94. Binary Tree Inorder Traversal
1. Question
Given the root of a binary tree, return the inorder traversal of its nodes' values.
2. Examples
Example 1:
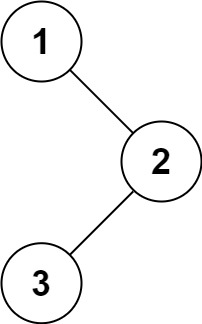
Input: root = [1,null,2,3]
Output: [1,3,2]
Example 2:
Input: root = []
Output: []
Example 3:
Input: root = [1]
Output: [1]
Example 4:
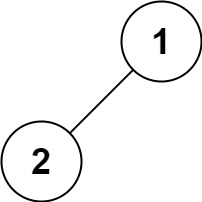
Input: root = [1,2]
Output: [2,1]
Example 5:
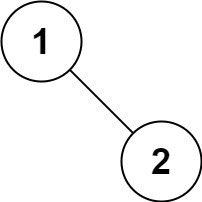
Input: root = [1,null,2]
Output: [1,2]
3. Constraints
- The number of nodes in the tree is in the range
[0, 100]
. -100 <= Node.val <= 100
Follow up: Recursive solution is trivial, could you do it iteratively?
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/binary-tree-inorder-traversal 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
class Solution {
List<Integer> list = new ArrayList<>();
public List<Integer> inorderTraversal(TreeNode root) {
sort(root);
return list;
}
public void sort(TreeNode root) {
if (root == null) {
return;
}
sort(root.left);
list.add(root.val);
sort(root.right);
}
}